React Native로 안드로이드 카카오 로그인을 구현해보겠습니다.
해당 게시글에서는 react-native-seoul/kakao-login 라이브러리를 사용합니다.
https://github.com/crossplatformkorea/react-native-kakao-login
GitHub - crossplatformkorea/react-native-kakao-login: react-native native module for Kakao sign in.
react-native native module for Kakao sign in. Contribute to crossplatformkorea/react-native-kakao-login development by creating an account on GitHub.
github.com
구현
1. 라이브러리 설치
npm install @react-native-seoul/kakao-login
2. Kakao developers 안드로이드 플랫폼 등록
Kakao developers에서 애플리케이션 생성 과정은 생략합니다. 카카오 계정 로그인 후 https://developers.kakao.com/console/app 에서 등록하시면 됩니다.
패키지명을 입력하고, 키 해시를 등록합니다.
기본 디버그 키 해시인 Xo8WBi6jzSxKDVR4drqm84yr9iU= 를 등록하면 됩니다.
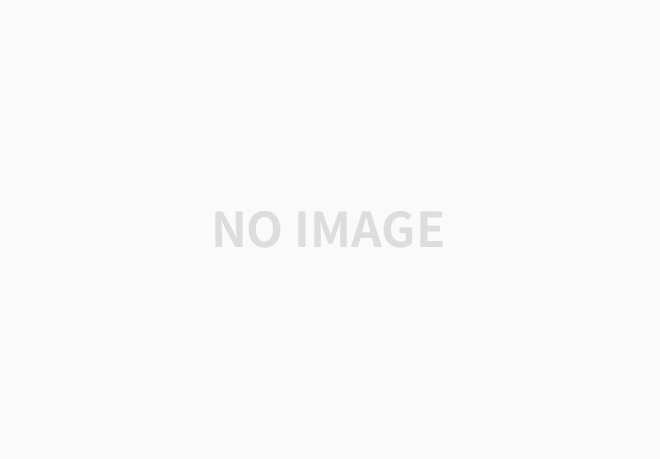
3. Kakao developers 카카오 로그인 활성화
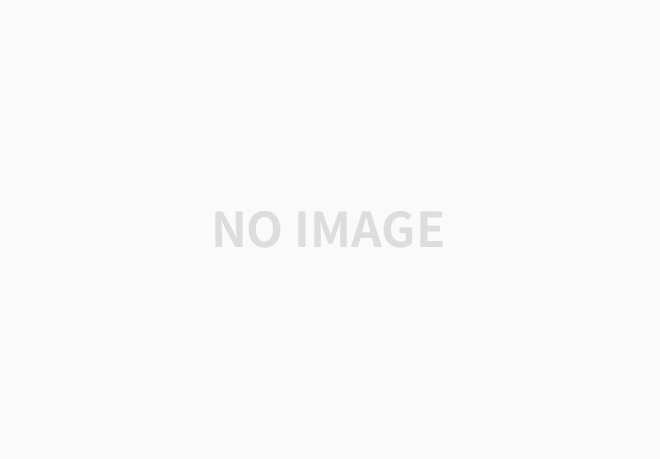
4. 네이티브 앱 키 복사
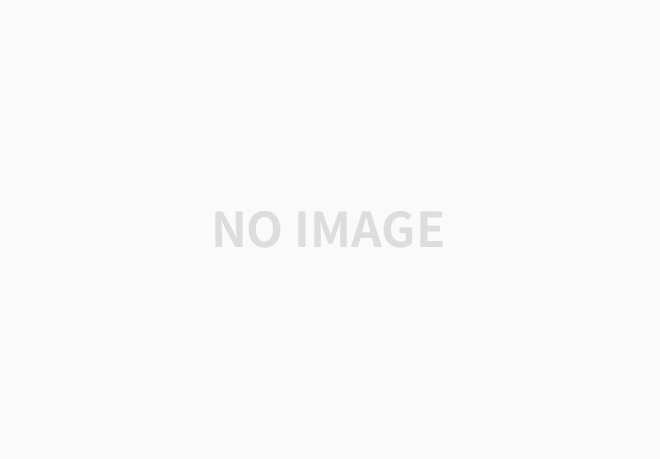
5. Redirect URI 설정
android/app/src/main/AndroidManifest.xml에 다음 코드를 추가합니다.
<activity
android:name="com.kakao.sdk.auth.AuthCodeHandlerActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<!-- Redirect URI: "kakao{NATIVE_APP_KEY}://oauth“ -->
<data android:host="oauth"
android:scheme="kakao{카카오 네이티브 앱 key를 입력해주세요}" />
</intent-filter>
</activity>
이때, { }도 제거해주세요. ex) android:scheme="kakaoaderrtdf..." />
중괄호를 제거하지 않으면 Confirm and Continue 버튼을 클릭해도 다음 단계로 넘어가지 않습니다.
android/app/src/main/res/values/strings.xml에 다음 코드를 추가합니다.
<resources>
<string name="app_name">KakaoLoginExample</string>
<string name="kakao_app_key">your_app_key</string> <!-- 추가할 코드 -->
</resources>
6. android/build.gradle에 Maven 추가
repositories {
google()
mavenCentral()
//추가할 코드
maven { url 'https://devrepo.kakao.com/nexus/content/groups/public/' }
}
7. 예제 코드 작성 후 실행 (App.tsx)
import { Pressable, ScrollView, StyleSheet, Text, View } from "react-native";
import React, { useState } from "react";
import {
login,
logout,
getProfile as getKakaoProfile,
shippingAddresses as getKakaoShippingAddresses,
unlink,
} from "@react-native-seoul/kakao-login";
const App = () => {
const [result, setResult] = useState<string>("");
const signInWithKakao = async (): Promise<void> => {
try {
const token = await login();
setResult(JSON.stringify(token));
console.log("login success ", token.accessToken);
} catch (err) {
console.error("login err", err);
}
};
const signOutWithKakao = async (): Promise<void> => {
try {
const message = await logout();
setResult(message);
} catch (err) {
console.error("signOut error", err);
}
};
const getProfile = async (): Promise<void> => {
try {
const profile = await getKakaoProfile();
setResult(JSON.stringify(profile));
} catch (err) {
console.error("signOut error", err);
}
};
const getShippingAddresses = async (): Promise<void> => {
try {
const shippingAddresses = await getKakaoShippingAddresses();
setResult(JSON.stringify(shippingAddresses));
} catch (err) {
console.error("signOut error", err);
}
};
const unlinkKakao = async (): Promise<void> => {
try {
const message = await unlink();
setResult(message);
} catch (err) {
console.error("signOut error", err);
}
};
return (
<View style={styles.container}>
<View style={styles.resultContainer}>
<ScrollView>
<Text>{result}</Text>
<View style={{ height: 100 }} />
</ScrollView>
</View>
<Pressable
style={styles.button}
onPress={() => {
signInWithKakao();
}}
>
<Text style={styles.text}>카카오 로그인</Text>
</Pressable>
<Pressable style={styles.button} onPress={() => getProfile()}>
<Text style={styles.text}>프로필 조회</Text>
</Pressable>
<Pressable style={styles.button} onPress={() => getShippingAddresses()}>
<Text style={styles.text}>배송주소록 조회</Text>
</Pressable>
<Pressable style={styles.button} onPress={() => unlinkKakao()}>
<Text style={styles.text}>링크 해제</Text>
</Pressable>
<Pressable style={styles.button} onPress={() => signOutWithKakao()}>
<Text style={styles.text}>카카오 로그아웃</Text>
</Pressable>
</View>
);
};
export default App;
const styles = StyleSheet.create({
container: {
height: "100%",
justifyContent: "flex-end",
alignItems: "center",
paddingBottom: 100,
},
resultContainer: {
flexDirection: "column",
width: "100%",
padding: 24,
},
button: {
backgroundColor: "#FEE500",
borderRadius: 40,
borderWidth: 1,
width: 250,
height: 40,
paddingHorizontal: 20,
paddingVertical: 10,
marginTop: 10,
},
text: {
textAlign: "center",
},
});
자세한 내용은 아래 링크를 참조하시면 됩니다.
react-native-kakao-login/KakaoLoginExample/src/pages/Intro.tsx at main · crossplatformkorea/react-native-kakao-login
react-native native module for Kakao sign in. Contribute to crossplatformkorea/react-native-kakao-login development by creating an account on GitHub.
github.com
terminal에 다음과 같이 작성하여 Android Emulator를 실행합니다.
npx react-native run-android
로그인 성공 시, 다음과 같은 Log가 출력됩니다.
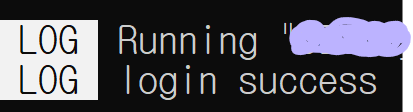
에러
1. Confirm and Continue 버튼 클릭 안됨
원인)
android/app/src/main/AndroidManifest.xml에 추가한 kakao{카카오 네이티드 앱 key} 부분에서 중괄호를 제거하지 않았을 경우 다음 단계로 넘어가지 않습니다.
해결)
android:scheme="kakaokakaonativeappkey"와 같이 중괄호를 제거해보세요.